本文最后更新于:2024-01-03T09:59:41+08:00
题目描述
这是 LeetCode
上的 2215.
找出两数组的不同 ,难度为简单。
给你两个下标从 0
开始的整数数组 nums1
和
nums2
,请你返回一个长度为 2
的列表
answer
,其中:
answer[0]
是 nums1
中所有
不 存在于 nums2
中的 不同
整数组成的列表。
answer[1]
是 nums2
中所有
不 存在于 nums1
中的 不同
整数组成的列表。
注意:列表中的整数可以按 任意
顺序返回。
示例 1:
1 2 3 4 5
| 输入:nums1 = [1,2,3], nums2 = [2,4,6] 输出:[[1,3],[4,6]] 解释: 对于 nums1 ,nums1[1] = 2 出现在 nums2 中下标 0 处,然而 nums1[0] = 1 和 nums1[2] = 3 没有出现在 nums2 中。因此,answer[0] = [1,3]。 对于 nums2 ,nums2[0] = 2 出现在 nums1 中下标 1 处,然而 nums2[1] = 4 和 nums2[2] = 6 没有出现在 nums2 中。因此,answer[1] = [4,6]。
|
示例 2:
1 2 3 4 5
| 输入:nums1 = [1,2,3,3], nums2 = [1,1,2,2] 输出:[[3],[]] 解释: 对于 nums1 ,nums1[2] 和 nums1[3] 没有出现在 nums2 中。由于 nums1[2] == nums1[3] ,二者的值只需要在 answer[0] 中出现一次,故 answer[0] = [3]。 nums2 中的每个整数都在 nums1 中出现,因此,answer[1] = [] 。
|
提示:
1 <= nums1.length, nums2.length <= 1000
-1000 <= nums1[i], nums2[i] <= 1000
解答
方法一:哈希表
根据题目的意思,先找出两个数组的交集,然后再分别遍历两个数组,找出与交集不同的数即可。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| class Solution { public List<List<Integer>> findDifference(int[] nums1, int[] nums2) { var tmp = new HashSet<Integer>(); for (int i = 0; i < nums1.length; ++i) { tmp.add(nums1[i]); } var hash = new HashSet<Integer>(); for (int i = 0; i < nums2.length; ++i) { if (tmp.contains(nums2[i])) { hash.add(nums2[i]); } } var set1 = new HashSet<Integer>(); for (int i = 0; i < nums1.length; ++i) { if (!hash.contains(nums1[i])) { set1.add(nums1[i]); } } var set2 = new HashSet<Integer>(); for (int i = 0; i < nums2.length; ++i) { if (!hash.contains(nums2[i])) { set2.add(nums2[i]); } } return Arrays.asList(new ArrayList<>(set1), new ArrayList<>(set2)); } }
|
方法二:数组模拟哈希表
用数组模拟哈希表,在遍历第二个数组的过程中就可以添加不在交集中的数,其基本思想和方法一一致。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| class Solution { public List<List<Integer>> findDifference(int[] nums1, int[] nums2) { List<List<Integer>> resList = new ArrayList<>(); List<Integer> res1 = new ArrayList<>(); List<Integer> res2 = new ArrayList<>(); int table[] = new int[2001]; for (int n : nums1){ table[n + 1000] = 1; } for (int n : nums2){ if (table[n + 1000] == 0) { res2.add(n); } table[n + 1000] = 2; } for (int n : nums1) { if(table[n + 1000] == 1) { res1.add(n); } table[n + 1000] = 2; } resList.add(res1); resList.add(res2); return resList; } }
|
每题一图
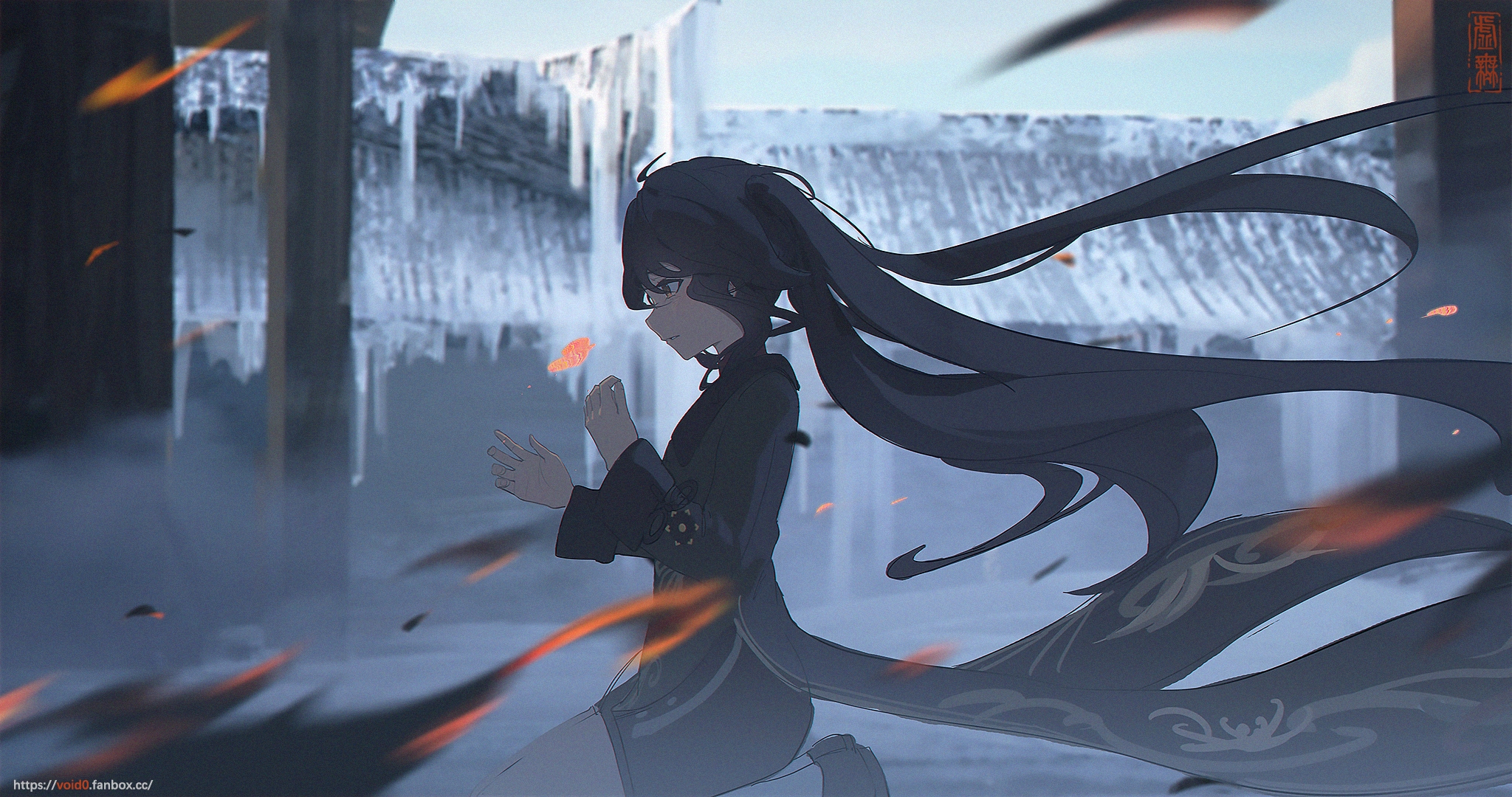